- 发布时间
Removebg API 使用文档
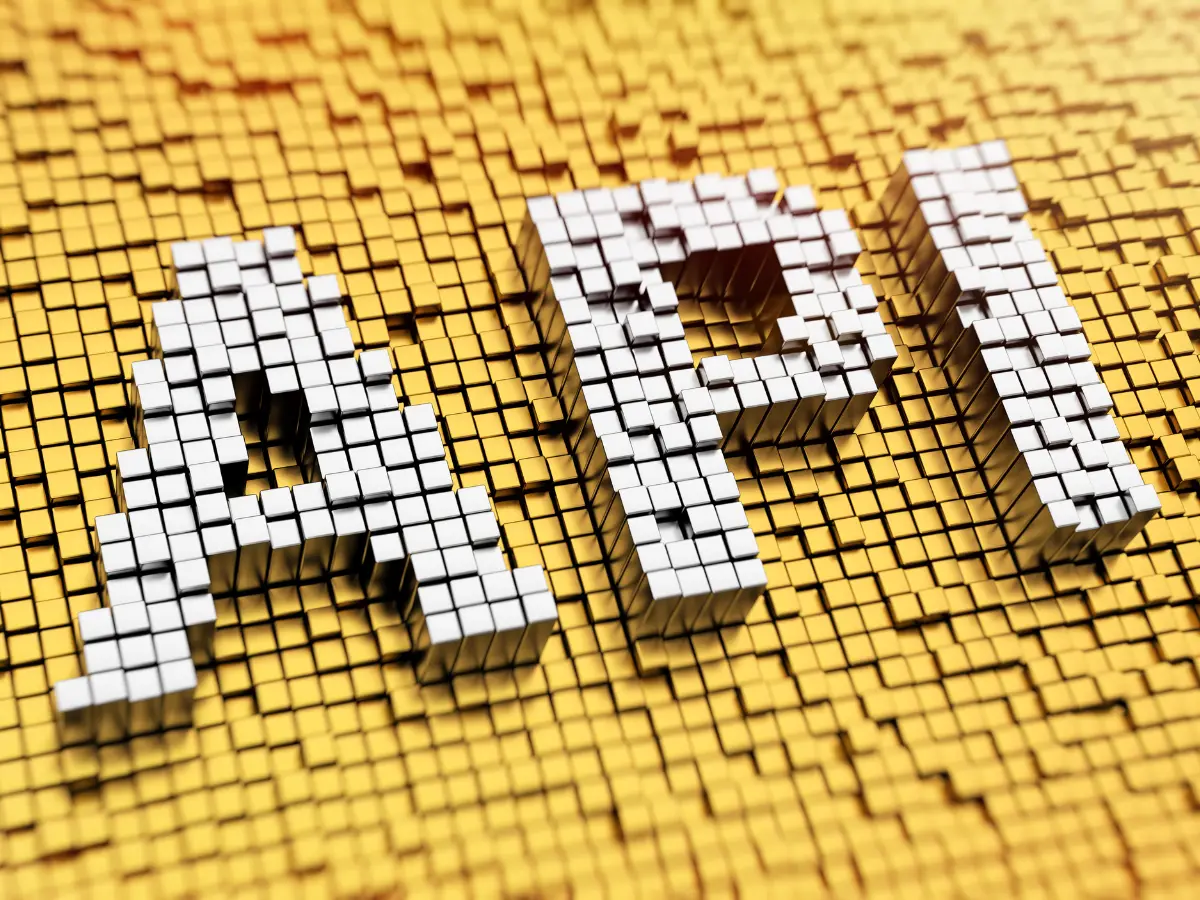
快速开始
curl --location 'https://removebg.one/api/v1/predict' \
--header 'Authorization: Bearer $API_KEY' \
--form 'file=@"test.png"'
请求域名
https://removebg.one
请求接口
/api/v1/predict
创建 API Key
在 API Key 页面生成或重置 API Key。
请求参数介绍
-
目前仅支持 formData 传递 File 对象,所以 Content-Type 请始终设置为 multipart/form-data。
-
在 API Key 页面生成 Key 后,将 Key 值设置到 Authorization 请求头。
Content-Type: multipart/form-data
Authorization: Bearer $API_KEY
请记得将 $API_KEY 替换为您的 API Key。
响应参数介绍
当 code 值返回 0 时表示接口调用成功,除此之外都是失败,具体失败原因在 message 字段中。
- 成功响应
{
"code": 0,
"data": {
"cutoutUrl": "https://r.removebg.one/static/8d062cc4-7d1d-4390-b958-a88e3a714e68.png?signature=...",
"maskUrl": "https://r.removebg.one/static/acd1904f-e7b6-4e20-92cc-29f19659caf8.png?signature=..."
}
}
- 失败响应
{
"code": 400,
"message": "Invalid request parameters."
}
请注意:成功后返回的图片地址仅 1 个小时有效,请根据您的业务及时保存或给出提示。
代码示例
Fetch
const formData = new FormData()
formData.append('file', File)
fetch('https://removebg.one/api/v1/predict', {
method: 'POST',
body: formData,
headers: {
Authorization: 'Bearer $API_KEY',
},
})
Java
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.FileBody;
import org.apache.http.util.EntityUtils;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
import java.io.File;
public class RemoveBgApiRequest {
public static void main(String[] args) throws Exception {
String apiKey = "YOUR_API_KEY"; // Replace with your API key
String filePath = "test.png"; // Replace with your file path
String url = "https://removebg.one/api/v1/predict";
File file = new File(filePath);
// Create HTTP client
try (CloseableHttpClient client = HttpClients.createDefault()) {
HttpPost post = new HttpPost(url);
post.setHeader("Authorization", "Bearer " + apiKey);
// Build multipart form data
FileBody fileBody = new FileBody(file);
HttpEntity entity = MultipartEntityBuilder.create()
.addPart("file", fileBody)
.build();
post.setEntity(entity);
// Execute request
String response = EntityUtils.toString(client.execute(post).getEntity());
// Parse the JSON response
JsonObject jsonResponse = JsonParser.parseString(response).getAsJsonObject();
int code = jsonResponse.get("code").getAsInt();
if (code == 0) {
// Success
System.out.println("Success: Response data = " + jsonResponse.getAsJsonObject("data"));
} else {
// Failure
String message = jsonResponse.has("message") ? jsonResponse.get("message").getAsString() : "Unknown error";
System.out.println("Failure: " + message);
}
}
}
}
Python
import requests
# Replace with your actual API key
api_key = 'your_api_key_here'
url = 'https://removebg.one/api/v1/predict'
# Path to the image file
file_path = 'test.png'
# Set up the headers with Authorization
headers = {
'Authorization': f'Bearer {api_key}'
}
# Open the image file in binary mode
with open(file_path, 'rb') as image_file:
# Send the POST request with the file
response = requests.post(url, headers=headers, files={'file': image_file})
# Check the response status
if response.code == 0:
print('Request successful')
# You can save or process the response content as needed
with open('output.png', 'wb') as output_file:
output_file.write(response.content)
else:
print(f'Error: {response.code}')
最后
若有问题,请发送邮件到 [email protected] 咨询。
- 作者
- 作者名称
- 官方
- 网站
- 在线抠图